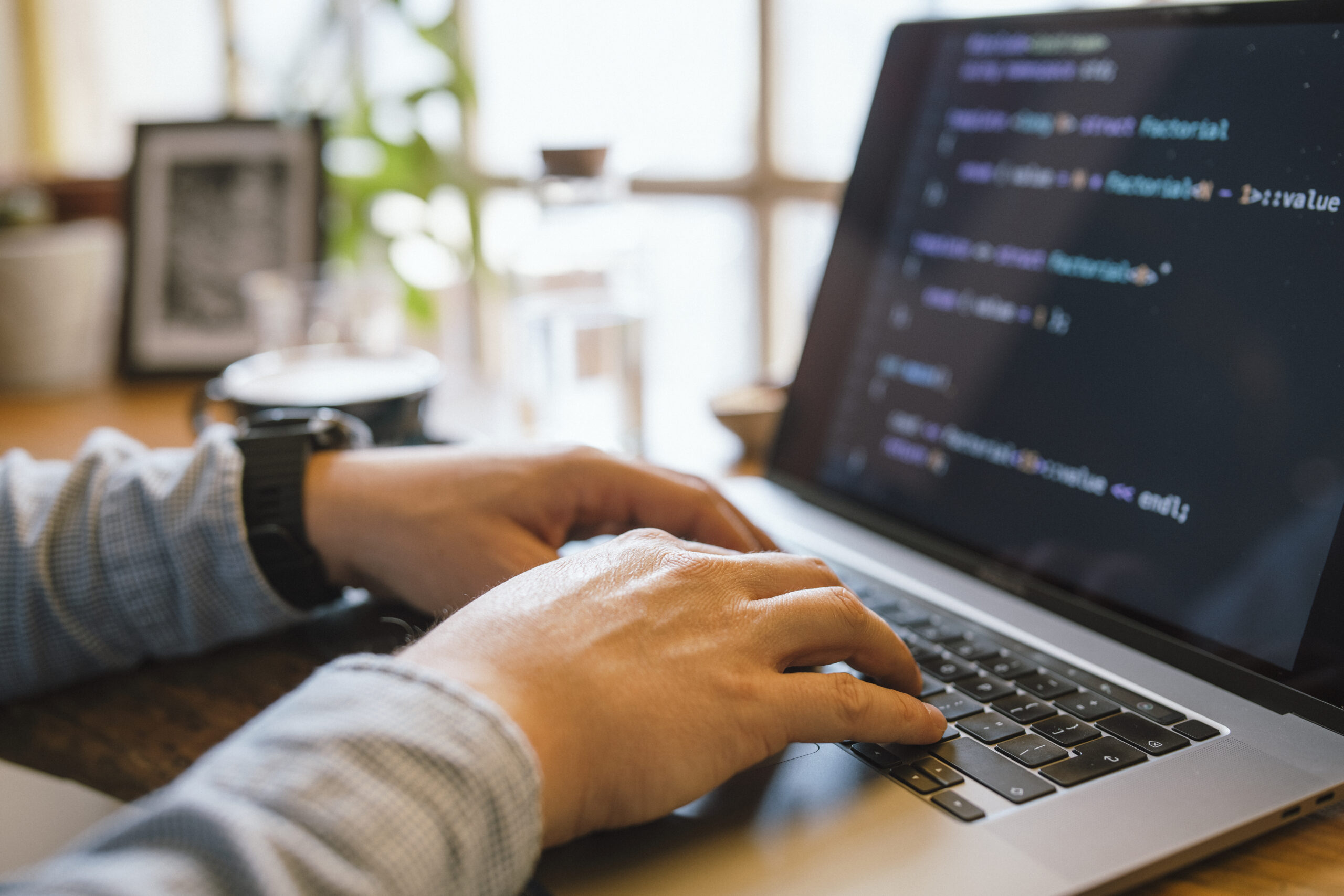
Debugging is Among the most critical — however typically forgotten — skills inside a developer’s toolkit. It isn't nearly repairing broken code; it’s about comprehension how and why points go Completely wrong, and Discovering to think methodically to solve problems efficiently. Whether or not you're a beginner or perhaps a seasoned developer, sharpening your debugging abilities can preserve hrs of disappointment and substantially increase your productiveness. Listed below are numerous methods to assist developers amount up their debugging video game by me, Gustavo Woltmann.
Grasp Your Equipment
One of many quickest means builders can elevate their debugging capabilities is by mastering the resources they use each day. While crafting code is just one Section of improvement, knowing ways to connect with it proficiently in the course of execution is equally vital. Present day improvement environments occur Outfitted with powerful debugging abilities — but several builders only scratch the floor of what these tools can perform.
Consider, for example, an Built-in Improvement Ecosystem (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications enable you to set breakpoints, inspect the value of variables at runtime, step by way of code line by line, as well as modify code on the fly. When made use of the right way, they Allow you to notice particularly how your code behaves for the duration of execution, which can be invaluable for monitoring down elusive bugs.
Browser developer equipment, such as Chrome DevTools, are indispensable for entrance-end developers. They allow you to inspect the DOM, watch network requests, watch real-time effectiveness metrics, and debug JavaScript in the browser. Mastering the console, resources, and community tabs can flip annoying UI issues into manageable jobs.
For backend or method-degree builders, applications like GDB (GNU Debugger), Valgrind, or LLDB supply deep Regulate over operating procedures and memory administration. Learning these resources can have a steeper learning curve but pays off when debugging efficiency difficulties, memory leaks, or segmentation faults.
Further than your IDE or debugger, turn out to be cozy with Model Regulate methods like Git to comprehend code history, discover the exact second bugs have been launched, and isolate problematic improvements.
Finally, mastering your tools implies heading over and above default options and shortcuts — it’s about establishing an personal familiarity with your progress natural environment to make sure that when challenges crop up, you’re not shed at the hours of darkness. The greater you are aware of your applications, the greater time you may shell out fixing the actual difficulty as opposed to fumbling by means of the process.
Reproduce the issue
Probably the most crucial — and often overlooked — ways in helpful debugging is reproducing the condition. In advance of jumping in to the code or making guesses, builders will need to make a steady atmosphere or scenario wherever the bug reliably appears. With out reproducibility, correcting a bug will become a recreation of chance, normally resulting in wasted time and fragile code variations.
Step one in reproducing a problem is accumulating as much context as feasible. Question queries like: What actions brought about the issue? Which ecosystem was it in — growth, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The more detail you've got, the easier it will become to isolate the exact ailments below which the bug takes place.
When you’ve gathered sufficient information and facts, make an effort to recreate the condition in your local ecosystem. This might necessarily mean inputting the identical details, simulating comparable consumer interactions, or mimicking system states. If The problem seems intermittently, contemplate crafting automated exams that replicate the sting cases or condition transitions included. These tests not merely assistance expose the issue and also prevent regressions Later on.
From time to time, The difficulty might be natural environment-unique — it might take place only on selected functioning methods, browsers, or beneath unique configurations. Applying tools like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms is often instrumental in replicating these types of bugs.
Reproducing the trouble isn’t only a action — it’s a mentality. It requires patience, observation, along with a methodical strategy. But as soon as you can continually recreate the bug, you might be now midway to correcting it. Which has a reproducible scenario, you can use your debugging tools much more successfully, check prospective fixes securely, and talk a lot more Obviously with the staff or end users. It turns an abstract grievance into a concrete challenge — Which’s where builders prosper.
Read through and Recognize the Error Messages
Error messages are often the most valuable clues a developer has when a little something goes Completely wrong. Rather then observing them as annoying interruptions, developers should master to take care of error messages as direct communications within the process. They typically let you know precisely what transpired, wherever it occurred, and occasionally even why it transpired — if you understand how to interpret them.
Commence by reading the information very carefully and in comprehensive. Quite a few developers, specially when beneath time stress, look at the primary line and instantly get started generating assumptions. But deeper from the error stack or logs may well lie the correct root cause. Don’t just duplicate and paste mistake messages into serps — go through and understand them 1st.
Break the error down into pieces. Could it be a syntax mistake, a runtime exception, or even a logic mistake? Does it position to a specific file and line variety? What module or function activated it? These questions can information your investigation and point you toward the liable code.
It’s also useful to be aware of the terminology from the programming language or framework you’re working with. Mistake messages in languages like Python, JavaScript, or Java generally adhere to predictable designs, and Discovering to recognize these can substantially increase your debugging procedure.
Some glitches are imprecise or generic, and in Individuals scenarios, it’s crucial to examine the context through which the mistake happened. Verify relevant log entries, enter values, and up to date modifications inside the codebase.
Don’t forget compiler or linter warnings possibly. These often precede bigger difficulties and supply hints about potential bugs.
In the end, error messages are usually not your enemies—they’re your guides. Studying to interpret them appropriately turns chaos into clarity, supporting you pinpoint difficulties a lot quicker, reduce debugging time, and become a much more productive and self-confident developer.
Use Logging Sensibly
Logging is The most highly effective applications inside a developer’s debugging toolkit. When employed properly, it offers true-time insights into how an software behaves, serving to you have an understanding of what’s happening under the hood without needing to pause execution or step through the code line by line.
A good logging strategy starts off with knowing what to log and at what amount. Typical logging ranges consist of DEBUG, INFO, Alert, Mistake, and Deadly. Use DEBUG for in depth diagnostic info throughout improvement, Information for basic gatherings (like thriving get started-ups), Alert for opportunity concerns that don’t split the applying, Mistake for true difficulties, and FATAL in the event the technique can’t proceed.
Stay away from flooding your logs with excessive or irrelevant info. A lot of logging can obscure essential messages and decelerate your process. Center on crucial events, point out alterations, input/output values, and critical decision details in the code.
Format your log messages clearly and continuously. Incorporate context, including timestamps, ask for IDs, and function names, so it’s much easier to trace troubles in distributed programs or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs let you observe how variables evolve, what conditions are satisfied, and what branches of logic are executed—all without halting This system. They’re Particularly precious in manufacturing environments wherever stepping via code isn’t doable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that assistance log rotation, filtering, and integration with checking dashboards.
In the end, intelligent logging is about stability and clarity. That has a effectively-assumed-out logging tactic, you are able to decrease the time it will take to identify challenges, acquire further visibility into your purposes, and improve the All round maintainability and trustworthiness of your code.
Feel Just like a Detective
Debugging is not just a technical process—it is a method of investigation. To successfully discover and take care of bugs, developers need to solution the process like a detective fixing a thriller. This mentality helps break down sophisticated difficulties into workable pieces and follow clues logically to uncover the root result in.
Commence by collecting evidence. Think about the indications of the problem: error messages, incorrect output, or overall performance problems. Much like a detective surveys a crime scene, gather as much related info as you may without the need of leaping to conclusions. Use logs, take a look at scenarios, and consumer studies to piece collectively a clear image of what’s happening.
Next, form hypotheses. Ask you: What can be producing this habits? Have any alterations just lately been created for the codebase? Has this problem happened in advance of underneath related situations? The goal is to slender down opportunities and determine possible culprits.
Then, test your theories systematically. Attempt to recreate the issue in a managed setting. Should you suspect a specific purpose or part, isolate it and verify if the issue persists. Just like a detective conducting interviews, inquire your code questions and Enable the outcome lead you nearer to the truth.
Pay back near interest to small aspects. Bugs generally conceal within the the very least anticipated sites—just like a missing semicolon, an off-by-just one error, or simply a race issue. Be thorough and individual, resisting the urge to patch The difficulty with no fully knowledge it. Temporary fixes may well conceal the actual issue, just for it to resurface later.
Last of all, continue to keep notes on That which you attempted and figured out. Equally as detectives log their investigations, documenting your debugging method can help you save time for long term troubles and help Other individuals have an understanding of your reasoning.
By considering just like a detective, builders can sharpen their analytical skills, strategy challenges methodically, and become more effective at uncovering hidden difficulties in complex methods.
Publish Checks
Crafting tests is one of the best strategies to help your debugging techniques and overall improvement effectiveness. Exams not merely enable capture bugs early but will also function a safety net that gives you self-assurance when producing alterations on your codebase. A perfectly-analyzed software is much easier to debug mainly because it helps check here you to pinpoint accurately where and when a problem occurs.
Get started with device tests, which concentrate on person functions or modules. These small, isolated checks can immediately expose irrespective of whether a selected bit of logic is Doing work as predicted. Each time a check fails, you instantly know exactly where to look, significantly lessening enough time put in debugging. Unit tests are Primarily practical for catching regression bugs—difficulties that reappear soon after Formerly being preset.
Upcoming, integrate integration tests and end-to-close assessments into your workflow. These aid make sure that various portions of your application work alongside one another easily. They’re especially practical for catching bugs that arise in complicated units with a number of components or products and services interacting. If anything breaks, your tests can inform you which Portion of the pipeline unsuccessful and beneath what conditions.
Producing tests also forces you to definitely think critically regarding your code. To test a element correctly, you would like to comprehend its inputs, envisioned outputs, and edge circumstances. This volume of knowing The natural way qualified prospects to raised code construction and less bugs.
When debugging an issue, composing a failing exam that reproduces the bug may be a strong starting point. After the take a look at fails consistently, it is possible to deal with repairing the bug and enjoy your test move when The problem is fixed. This method makes sure that a similar bug doesn’t return in the future.
In a nutshell, producing checks turns debugging from a aggravating guessing video game right into a structured and predictable process—serving to you capture more bugs, more quickly plus much more reliably.
Choose Breaks
When debugging a tricky problem, it’s straightforward to be immersed in the situation—gazing your screen for hours, attempting Remedy soon after Option. But One of the more underrated debugging tools is simply stepping away. Taking breaks helps you reset your mind, decrease aggravation, and often see the issue from a new perspective.
When you're too close to the code for too long, cognitive exhaustion sets in. You might start overlooking noticeable faults or misreading code that you choose to wrote just several hours before. With this condition, your brain turns into significantly less effective at issue-solving. A brief stroll, a coffee break, or simply switching to another undertaking for 10–15 minutes can refresh your focus. Lots of builders report locating the root of a problem when they've taken time and energy to disconnect, letting their subconscious work during the qualifications.
Breaks also aid stop burnout, Primarily through more time debugging sessions. Sitting down in front of a screen, mentally caught, is not just unproductive but also draining. Stepping absent permits you to return with renewed energy and also a clearer attitude. You may quickly recognize a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded you prior to.
For those who’re caught, a very good guideline is to established a timer—debug actively for forty five–60 minutes, then have a 5–ten moment split. Use that point to move around, stretch, or do a little something unrelated to code. It might experience counterintuitive, Specially less than restricted deadlines, but it really truly causes more quickly and more practical debugging in the long run.
Briefly, having breaks just isn't an indication of weakness—it’s a smart tactic. It gives your brain Place to breathe, increases your viewpoint, and will help you steer clear of the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and rest is a component of resolving it.
Discover From Every single Bug
Each individual bug you encounter is much more than simply A short lived setback—it's an opportunity to expand for a developer. Whether it’s a syntax error, a logic flaw, or even a deep architectural challenge, every one can instruct you something beneficial in case you make the effort to reflect and evaluate what went Mistaken.
Start out by inquiring you a few important concerns after the bug is settled: What triggered it? Why did it go unnoticed? Could it are already caught previously with superior tactics like device tests, code opinions, or logging? The responses generally expose blind spots with your workflow or comprehension and allow you to Create more robust coding practices relocating forward.
Documenting bugs may also be a great habit. Keep a developer journal or maintain a log in which you Observe down bugs you’ve encountered, the way you solved them, and Whatever you realized. As time passes, you’ll start to see styles—recurring troubles or frequent blunders—that you could proactively avoid.
In workforce environments, sharing That which you've uncovered from a bug with all your friends could be Particularly powerful. Irrespective of whether it’s by way of a Slack message, a brief publish-up, or a quick know-how-sharing session, supporting Other people steer clear of the very same problem boosts workforce effectiveness and cultivates a stronger Mastering tradition.
More importantly, viewing bugs as classes shifts your mindset from annoyance to curiosity. As opposed to dreading bugs, you’ll start appreciating them as necessary elements of your progress journey. In any case, some of the ideal developers will not be those who compose fantastic code, but people who consistently find out from their issues.
Ultimately, Each individual bug you resolve provides a brand new layer on your skill set. So upcoming time you squash a bug, have a second to mirror—you’ll occur away a smarter, far more able developer due to it.
Conclusion
Increasing your debugging abilities normally takes time, observe, and patience — even so the payoff is large. It makes you a more productive, self-assured, and able developer. The next time you are knee-deep in the mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to become greater at That which you do.